How to develop Scalable Programs to be a Developer By Gustavo Woltmann
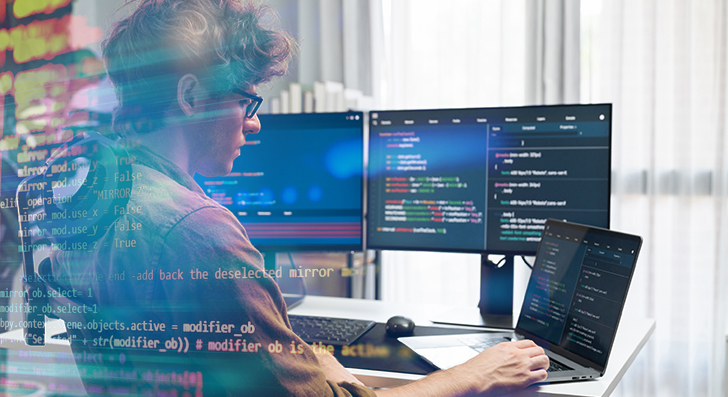
Scalability means your application can deal with growth—extra people, a lot more information, and even more traffic—without the need of breaking. As being a developer, building with scalability in your mind saves time and strain later on. Here’s a transparent and sensible guideline to help you start out by Gustavo Woltmann.
Design and style for Scalability from the beginning
Scalability isn't really something you bolt on later on—it should be portion of one's system from the start. Numerous apps fail if they develop rapid since the first style can’t deal with the additional load. As a developer, you must Imagine early about how your process will behave under pressure.
Start off by building your architecture for being flexible. Keep away from monolithic codebases where by every little thing is tightly related. Instead, use modular layout or microservices. These styles break your app into lesser, independent areas. Each individual module or services can scale on its own devoid of influencing the whole program.
Also, contemplate your database from day one particular. Will it have to have to handle a million consumers or merely 100? Pick the right variety—relational or NoSQL—dependant on how your data will develop. Prepare for sharding, indexing, and backups early, Even though you don’t will need them nonetheless.
Another essential level is to stay away from hardcoding assumptions. Don’t write code that only works below present situations. Think of what would come about If the consumer foundation doubled tomorrow. Would your application crash? Would the database decelerate?
Use layout designs that help scaling, like concept queues or occasion-driven systems. These help your app take care of a lot more requests without having acquiring overloaded.
Once you Develop with scalability in mind, you are not just making ready for achievement—you are cutting down long run complications. A effectively-planned system is less complicated to keep up, adapt, and expand. It’s far better to prepare early than to rebuild later.
Use the correct Database
Deciding on the appropriate database is a vital Component of constructing scalable applications. Not all databases are crafted the same, and utilizing the Mistaken one can gradual you down as well as result in failures as your app grows.
Start out by comprehension your information. Is it really structured, like rows in the table? If Of course, a relational database like PostgreSQL or MySQL is a superb in shape. They're strong with associations, transactions, and consistency. In addition they assist scaling techniques like examine replicas, indexing, and partitioning to handle additional site visitors and details.
Should your details is much more adaptable—like consumer exercise logs, solution catalogs, or files—think about a NoSQL solution like MongoDB, Cassandra, or DynamoDB. NoSQL databases are much better at dealing with large volumes of unstructured or semi-structured knowledge and will scale horizontally much more easily.
Also, take into account your read and compose designs. Are you carrying out many reads with fewer writes? Use caching and browse replicas. Will you be managing a hefty write load? Explore databases which will handle large publish throughput, or simply event-primarily based knowledge storage units like Apache Kafka (for temporary facts streams).
It’s also good to think ahead. You may not want State-of-the-art scaling options now, but choosing a database that supports them indicates you won’t want to modify afterwards.
Use indexing to hurry up queries. Stay clear of unnecessary joins. Normalize or denormalize your information based on your accessibility patterns. And often check database efficiency when you improve.
To put it briefly, the ideal databases will depend on your application’s framework, pace demands, And the way you count on it to expand. Acquire time to choose properly—it’ll conserve lots of difficulty later.
Improve Code and Queries
Rapid code is vital to scalability. As your app grows, each small delay provides up. Inadequately prepared code or unoptimized queries can slow down performance and overload your procedure. That’s why it’s imperative that you Make productive logic from the start.
Get started by producing clear, uncomplicated code. Keep away from repeating logic and remove just about anything unwanted. Don’t select the most complex Option if an easy 1 is effective. Maintain your functions small, concentrated, and simple to check. Use profiling equipment to locate bottlenecks—sites in which your code takes far too extended to operate or employs an excessive amount of memory.
Upcoming, check out your database queries. These generally slow points down over the code alone. Ensure Each individual query only asks for the info you actually need to have. Avoid SELECT *, which fetches almost everything, and instead decide on unique fields. Use indexes to speed up lookups. And prevent performing too many joins, Primarily across massive tables.
For those who discover the same info staying requested repeatedly, use caching. Retail outlet the effects temporarily making use of applications like Redis or Memcached so you don’t must repeat high priced functions.
Also, batch your databases operations any time you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and helps make your application additional efficient.
Remember to check with huge datasets. Code and queries that operate great with a hundred information may possibly crash if they have to take care of one million.
To put it briefly, scalable applications are fast apps. Keep your code tight, your queries lean, and use caching when required. These actions aid your application remain clean and responsive, at the same time as the load boosts.
Leverage Load Balancing and Caching
As your application grows, it's to manage additional people plus much more targeted visitors. If all the things goes as a result of a person server, it will eventually immediately turn into a bottleneck. That’s where load balancing and caching come in. Both of these instruments enable maintain your app quick, stable, and scalable.
Load balancing spreads incoming targeted visitors throughout a number of servers. As an alternative to a single server carrying out all of the perform, the load balancer routes consumers to various servers based on availability. This suggests no solitary server gets overloaded. If one server goes down, the load balancer can mail visitors to the Other people. Tools like Nginx, HAProxy, or cloud-centered remedies from AWS and Google Cloud make this simple to set up.
Caching is about storing information quickly so it could be reused rapidly. When buyers ask for exactly the same information and facts yet again—like a product web site or possibly a profile—you don’t have to fetch it within the database every time. It is possible to provide it from the cache.
There's two typical different types of caching:
1. Server-facet caching (like Redis or Memcached) retailers data in memory for rapidly access.
2. Shopper-aspect caching (like browser caching or CDN caching) suppliers static information near the consumer.
Caching reduces database load, increases speed, and would make your app far more efficient.
Use caching for things that don’t improve usually. And generally make certain your cache is up-to-date when data does adjust.
To put it briefly, load balancing and caching are uncomplicated but powerful equipment. Alongside one another, they help your app cope with more end users, continue to be fast, and Recuperate from challenges. If you propose to develop, you may need both of those.
Use Cloud and Container Resources
To create scalable apps, you need resources that allow your app improve conveniently. That’s exactly where cloud platforms and containers are available in. They provide you overall flexibility, cut down set up time, and make scaling much smoother.
Cloud platforms like Amazon Net Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to rent servers and solutions as you will need them. You don’t really need to obtain components or guess upcoming potential. When traffic increases, you can include much more sources with only a few clicks or immediately utilizing automobile-scaling. When visitors drops, you may scale down to save cash.
These platforms also offer you companies like managed databases, storage, load balancing, and protection equipment. You'll be able to give attention to creating your app instead of running infrastructure.
Containers are A further critical Resource. A container deals your app and everything it really should operate—code, libraries, configurations—into one particular unit. This makes it quick to maneuver your app between environments, from a laptop computer towards the cloud, without surprises. Docker is the preferred Device for this.
When your application employs numerous containers, tools like Kubernetes make it easier to deal with them. Kubernetes handles deployment, scaling, and Restoration. If 1 section of your respective app crashes, it restarts it quickly.
Containers also ensure it is easy to individual elements of your application into providers. You can update or scale sections independently, which can be perfect for functionality and trustworthiness.
In a nutshell, using cloud and container instruments indicates you are able to scale rapid, deploy effortlessly, and Get well rapidly when challenges occur. In order for you your app to increase without boundaries, get started making use of these instruments early. They save time, lessen hazard, and enable you to continue to be focused on creating, not repairing.
Check Anything
In the event you don’t check your software, you received’t know when issues go Mistaken. Checking helps you see how your app is doing, location issues early, and make far better selections as your application grows. It’s a vital A part of creating scalable programs.
Get started by monitoring simple metrics like CPU utilization, memory, disk Place, and reaction time. Gustavo Woltmann blog These show you how your servers and services are performing. Equipment like Prometheus, Grafana, Datadog, or New Relic may help you accumulate and visualize this details.
Don’t just monitor your servers—monitor your app as well. Keep watch over how long it will take for consumers to load webpages, how often mistakes take place, and in which they take place. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s going on within your code.
Put in place alerts for significant challenges. One example is, If the reaction time goes previously mentioned a limit or perhaps a service goes down, you should get notified immediately. This allows you take care of difficulties rapidly, typically just before customers even notice.
Checking is likewise valuable if you make alterations. Should you deploy a new aspect and find out a spike in mistakes or slowdowns, you can roll it again ahead of it leads to real problems.
As your app grows, traffic and facts boost. With out checking, you’ll skip indications of difficulties till it’s much too late. But with the best tools set up, you stay on top of things.
In brief, monitoring will help you keep your application dependable and scalable. It’s not almost spotting failures—it’s about knowledge your method and ensuring that it works very well, even under pressure.
Ultimate Thoughts
Scalability isn’t just for significant organizations. Even compact apps will need a strong Basis. By building very carefully, optimizing sensibly, and using the appropriate tools, you'll be able to Establish apps that mature easily with no breaking stressed. Commence smaller, think huge, and Make intelligent.